What is Cow in Rust
Rust optimizes performance and efficiency with a diverse range of techniques. In this post, we'll explore one such technique: Copy-On-Write (COW).
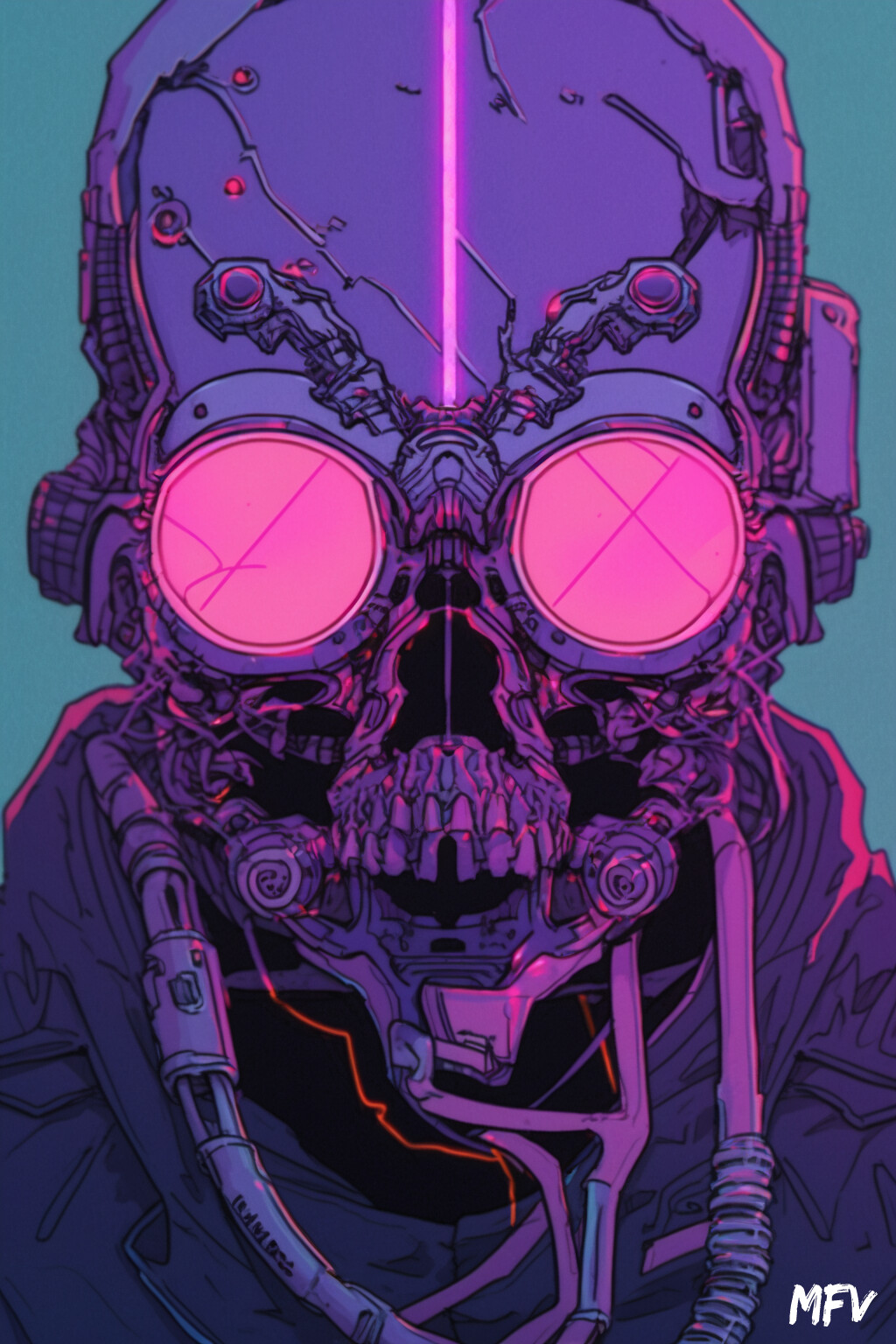
Cow is an enum that stands for Clone-on-Write. It can be used as a smart pointer for dealing with borrowed or owned values. This type can help us to avoid unnecessary memory allocation. In this post, I will explain how works, when to use it and why to use it.
How Cow work
Cow have two variants:
- Borrowed: Holds a Borrowed reference to data
- Owned: Holds an owned value
When a Cow instance is represented by the Borrowed variant, this means that the cow instance does not own the data and cannot modify it, it provides a read-only view of the data. In the other hand, if the Cow instance is represented by the Owned variant, this means the Cow instance owns the data and can modify it. However, the data is not immediately copied; instead, it is only copied when a modification is attempted.
When use Cow
One of the most common use cases for Cow is when you're working with strings. Here's a practical example to illustrate this. Let's say you have a program that needs to convert certain strings to uppercase, but you know that most of the time the strings will already be in uppercase. In this case, you can use Cow to avoid unnecessary allocations and copying of data.
fn to_uppercase(s: &str) -> Cow<str> {
if s.chars().any(|c| c.is_lowercase()){
Cow::Owned(s.to_uppercase())
}else{
Cow::Borrowed(s)
}
}
Why use Cow
Cow allows for efficient and flexible ownership of data without needing to make unnecessary copies. This help to avoid unnecessary memory allocations.
fn to_uppercase_one(s: &str) -> Cow<str> {
if s.chars().any(|c| c.is_lowercase()){
Cow::Owned(s.to_uppercase()) // <-- Allocation
}else{
Cow::Borrowed(s)
}
}
fn to_uppercase_two(s: &str) -> String {
if s.chars().any(|c| c.is_lowercase()){
s.to_uppercase() // <-- allocation
}else{
s.to_string() // <-- allocation
}
}
In the to_uppercase_one function, an allocation occurs when the Cow::Owned variant is created. This creates a new owned string that is a copy of the original string converted to uppercase, but only if the original string s is in lowercase. If the original string is already in uppercase, the function simply borrows the original string without making a copy.
In contrast, the to_uppercase_two function always creates a new owned string every time it's called, so an allocation occurs every time the function is called.
The advantage of to_uppercase_one is that it avoids unnecessary allocations and copying of data by borrowing the original string when possible. This can help improve the performance of your Rust program when you're working with large strings that don't need to be modified.
However, if you need to modify the string, or if the original string is always lowercase, using to_uppercase_two may be more appropriate. This function always creates an owned copy of the string and guarantees that the result will be in uppercase.
Conclusion
We can say that the Cow type can be thought of as a form of optional ownership. Provide a flexible way to work with values that may or may not need to be owned or modified, without requiring unnecessary copies or allocations, turning or software more memory-efficient.